Thursday, December 31, 2009
深入理解Javascript闭包(closure)
最近在网上查阅了不少Javascript闭包(closure)相关的资料,写的大多是非常的学术和专业。对于初学者来说别说理解闭包了,就连文字叙述都很难看懂。撰写此文的目的就是用最通俗的文字揭开Javascript闭包的真实面目。
一、什么是闭包?
“官方”的解释是:所谓“闭包”,指的是一个拥有许多变量和绑定了这些变量的环境的表达式(通常是一个函数),因而这些变量也是该表达式的一部分。
相信很少有人能直接看懂这句话,因为他描述的太学术。我想用如何在Javascript中创建一个闭包来告诉你什么是闭包,因为跳过闭包的创建过程直接理解闭包的定义是非常困难的。看下面这段代码:
function a(){
var i=0;
function b(){
alert(++i);
}
return b;
}
var c = a();
c();
这段代码有两个特点:
1、函数b嵌套在函数a内部;
2、函数a返回函数b。
这样在执行完var c=a()后,变量c实际上是指向了函数b,再执行c()后就会弹出一个窗口显示i的值(第一次为1)。这段代码其实就创建了一个闭包,为什么?因为函数a外的变量c引用了函数a内的函数b,就是说:
当函数a的内部函数b被函数a外的一个变量引用的时候,就创建了一个闭包。
我猜想你一定还是不理解闭包,因为你不知道闭包有什么作用,下面让我们继续探索。
二、闭包有什么作用?
简而言之,闭包的作用就是在a执行完并返回后,闭包使得Javascript的垃圾回收机制GC不会收回a所占用的资源,因为a的内部函数b的执行需要依赖a中的变量。这是对闭包作用的非常直白的描述,不专业也不严谨,但大概意思就是这样,理解闭包需要循序渐进的过程。
在上面的例子中,由于闭包的存在使得函数a返回后,a中的i始终存在,这样每次执行c(),i都是自加1后alert出i的值。
那 么我们来想象另一种情况,如果a返回的不是函数b,情况就完全不同了。因为a执行完后,b没有被返回给a的外界,只是被a所引用,而此时a也只会被b 引 用,因此函数a和b互相引用但又不被外界打扰(被外界引用),函数a和b就会被GC回收。(关于Javascript的垃圾回收机制将在后面详细介绍)
三、闭包内的微观世界
如 果要更加深入的了解闭包以及函数a和嵌套函数b的关系,我们需要引入另外几个概念:函数的执行环境 (excution context)、活动对象(call object)、作用域(scope)、作用域链(scope chain)。以函数a从定义到执行的过程为例阐述这几个概念。
1、当定义函数a的时候,js解释器会将函数a的作用域链(scope chain)设置为定义a时a所在的“环境”,如果a是一个全局函数,则scope chain中只有window对象。
2、当函数a执行的时候,a会进入相应的执行环境(excution context)。
3、在创建执行环境的过程中,首先会为a添加一个scope属性,即a的作用域,其值就为第1步中的scope chain。即a.scope=a的作用域链。
4、然后执行环境会创建一个活动对象(call object)。活动对象也是一个拥有属性的对象,但它不具有原型而且不能通过JavaScript代码直接访问。创建完活动对象后,把活动对象添加到a的作用域链的最顶端。此时a的作用域链包含了两个对象:a的活动对象和window对象。
5、下一步是在活动对象上添加一个arguments属性,它保存着调用函数a时所传递的参数。
6、最后把所有函数a的形参和内部的函数b的引用也添加到a的活动对象上。在这一步中,完成了函数b的的定义,因此如同第3步,函数b的作用域链被设置为b所被定义的环境,即a的作用域。
到此,整个函数a从定义到执行的步骤就完成了。此时a返回函数b的引用给c,又函数b的作用域链包含了对函数a的活动对象的引用,也就是说b可以访问到a中定义的所有变量和函数。函数b被c引用,函数b又依赖函数a,因此函数a在返回后不会被GC回收。
当函数b执行的时候亦会像以上步骤一样。因此,执行时b的作用域链包含了3个对象:b的活动对象、a的活动对象和window对象,如下图所示:
如图所示,当在函数b中访问一个变量的时候,搜索顺序是先搜索自身的活动对象,如果存在则返回,如果不存在将继续搜索函数a的活动对象,依 次查找,直到找到为止。如果整个作用域链上都无法找到,则返回undefined。如果函数b存在prototype原型对象,则在查找完自身的活动对象 后先查找自身的原型对象,再继续查找。这就是Javascript中的变量查找机制。
四、闭包的应用场景
1、保护函数内的变量安全。以最开始的例子为例,函数a中i只有函数b才能访问,而无法通过其他途径访问到,因此保护了i的安全性。
2、在内存中维持一个变量。依然如前例,由于闭包,函数a中i的一直存在于内存中,因此每次执行c(),都会给i自加1。
以上两点是闭包最基本的应用场景,很多经典案例都源于此。
五、Javascript的垃圾回收机制
在Javascript中,如果一个对象不再被引用,那么这个对象就会被GC回收。如果两个对象互相引用,而不再被第3者所引用,那么这两个互相引用的对象也会被回收。因为函数a被b引用,b又被a外的c引用,这就是为什么函数a执行后不会被回收的原因。
Monday, December 28, 2009
架构模式的介绍
几种典型的架构模式:
在开发系统软件时多用架构模式:
1、分层(Layer):从不同的层次来观察系统,处理不同层次问题的对象被封装到不同的层中。
2、管道和过滤器(Pipes and Filters):用数据流的观点来观察系统。整个系统由一些管道和过滤器组成,需要处理的数据同管道传送给每一个过滤器,每个过滤器就是一个处理步骤。每个过滤器可以单独修改,功能单一,并且它们之间的顺序可以进行配置。但数据通过了所有的过滤器后,就完成了所有的处理操作,得到了最终的处理结果。
一个典型的管道/过滤器体系结构的例子是以Unix shell编写的程序。Unix既提供一种符号,以连接各组成部分(Unix的进程),又提供某种进程运行时机制以实现管道。另一个著名的例子是传统的编译器。传统的编译器一直被认为是一种管道系统,在该系统中,一个阶段(包括词法分析、语法分析、语义分析和代码生成)的输出是另一个阶段的输入。
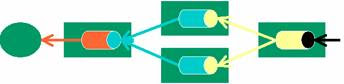
3、黑板(Blackboard):在这种架构中,有两种不同的构件:一种是表示当前状态中心数据结构;另一种是一种相互独立的构件,这些构件对中心数据进行操作。这种架构主要用于数据库和人工智能系统的开发。
在开发分布式软件时多用架构模式:
1、经纪人(Broker):客户和服务器通过一个经纪人部件进行通信,经纪人负责协调客户和服务器之间的操作,并且为客户和服务器发送请求和结果信息。
2、客户/服务器(Client/Server):系统分为客户和服务器,服务器一直处于侦听的状态,客户主动连接服务器,每个服务器可以为多个客户服务
3、点对点(Peer to Peer):系统中的节点都处于平等的地位,每个节点都可以连接其他节点。在这种架构中,一般需要由一个中心服务器完成发现和管理节点的操作。ICQ以及Web Service技术的大多数应用,都是典型的点对点结构。
开发交互软件时多用架构模式:
1、模型-视图-控制器(Model-View-Controller):当应用程序的用户界面非常复杂,且关于用户界面的需求很容易变化时,我们可以把交互类型的软件抽象成模型、视图和控制器这三类组件单元,这种抽象可以很好地分离用户界面和业务逻辑,适应变化的需求。大多数现代交互软件都在一定程度上符合这一架构模型的特点。
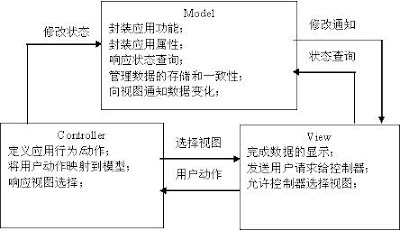
2、显示-抽象-控制(Presentation-Abstraction-COntrol):这是MVC的另一种变形。
http://en.wikipedia.org/wiki/Presentation-abstraction-control
软件的架构与设计模式之模式的种类介绍
由于[GOF95]是论述软件模式的著作的第一本,也是OO设计理论著作中最流行的一本,因此有些人常常使用设计模式(Design Pattern)一词来指所有直接处理软件的架构、设计、程序实现的任何种类的模式。另外一些人则强调要划分三种不同层次的模式:架构模式(Architectural Pattern)、设计模式(Design Pattern)、成例(Idiom)。成例有时称为代码模式(Coding Pattern)。
这三者之间的区别在于三种不同的模式存在于它们各自的抽象层次和具体层次上。架构模式是一个系统的高层次策略,涉及到大尺度的组件以及整体性质和力学。架构模式的好坏可以影响到总体布局和框架性结构。设计模式是中等尺度的结构策略。这些中等尺度的结构实现了一些大尺度组件的行为和它们之间的关系。模式的好坏不会影响到系统的总体布局和总体框架。设计模式定义出子系统或组件的微观结构。代码模式(或成例)是特定的范例和与特定语言有关的编程技巧。代码模式的好坏会影响到一个中等尺度组件的内部、外部的结构或行为的底层细节,但不会影响到一个部件或子系统的中等尺度的结构,更不会影响到系统的总体布局和大尺度框架。
代码模式或成例(Coding Pattern 或 Idiom)
代码模式(或成例)是较低层次的模式,并与编程语言密切相关。代码模式描述怎样利用一个特定的编程语言的特点来实现一个组件的某些特定的方面或关系。
较为著名的代码模式的例子包括双检锁(Double-Check Locking)模式等。
设计模式(Design Pattern)
一个设计模式提供一种提炼子系统或软件系统中的组件的,或者它们之间的关系的纲要设计。设计模式描述普遍存在的在相互通讯的组件中重复出现的结构,这种结构解决在一定的背景中的具有一般性的设计问题。
设计模式常常划分成不同的种类,常见的种类有:
创建型设计模式,如工厂方法(Factory Method)模式、抽象工厂(Abstract Factory)模式、原型(Prototype)模式、单例(Singleton)模式,建造(Builder)模式等
结构型设计模式,如合成(Composite)模式、装饰(Decorator)模式、代理(Proxy)模式、享元(Flyweight)模式、门面(Facade)模式、桥梁(Bridge)模式等
行为型模式,如模版方法(Template Method)模式、观察者(Observer)模式、迭代子(Iterator)模式、责任链(Chain of Responsibility)模式、备忘录(Memento)模式、命令(Command)模式、状态(State)模式、访问者(Visitor)模式等等。
以上是三种经典类型,实际上还有很多其他的类型,比如Fundamental型、Partition型,Relation型等等。设计模式在特定的编程语言中实现的时候,常常会用到代码模式。比如单例(Singleton)模式的实现常常涉及到双检锁(Double-Check Locking)模式等。
架构模式(Architectural Pattern)
一个架构模式描述软件系统里的基本的结构组织或纲要。架构模式提供一些事先定义好的子系统,指定它们的责任,并给出把它们组织在一起的法则和指南。有些作者把这种架构模式叫做系统模式[STELTING02]。
一个架构模式常常可以分解成很多个设计模式的联合使用。显然,MVC模式就是属于这一种模式。MVC模式常常包括调停者(Mediator)模式、策略(Strategy)模式、合成(Composite)模式、观察者(Observer)模式等。
此外,常见的架构模式还有:
•Layers(分层)模式,有时也称Tiers模式
•Blackboard(黑板)模式
•Broker(中介)模式
•Distributed Process(分散过程)模式
•Microkernel(微核)模式
架构模式常常划分成如下的几种:
一、 From Mud to Structure型。帮助架构师将系统合理划分,避免形成一个对象的海洋(A sea of objects)。包括Layers(分层)模式、Blackboard(黑板)模式、Pipes/Filters(管道/过滤器)模式等。
二、分散系统(Distributed Systems)型。为分散式系统提供完整的架构设计,包括像Broker(中介)模式等。
三、人机互动(Interactive Systems)型,支持包含有人机互动介面的系统的架构设计,例子包括MVC(Model-View-Controller)模式、PAC(Presentation-Abstraction-Control)模式等。
四、Adaptable Systems型,支持应用系统适应技术的变化、软件功能需求的变化。如Reflection(反射)模式、Microkernel(微核)模式等。
Thursday, December 17, 2009
在Java中,什么是Overriding?什么是Overloading?
a and b: 10 20
Inside test(double) a: 88
Inside test(double) a: 123.2
HTTP协议通信过程
HTTP协议通信过程
当我们在浏览器的地址栏输入“www.baidu.com”然后按回车,这之后发生了什么事,我们直接看到的是打开了对应的网页,那么内部客户端和服务端是如何通信的呢?
1、 1、URL自动解析
HTTP URL包含了用于查找某个资源的足够信息,基本格式如下:HTTP://host[“:”port] [abs_path],其中HTTP表示桶盖HTTP协议来定位网络资源;host表示合法的主机域名或IP地址,port指定一个端口号,缺省 80;abs_path指定请求资源的URI;如果URL中没有给出abs_path,那么当它作为请求URI时,必须以“/”的形式给出,通常这个工作浏览器自动帮我们完成。
例如:输入www.163.com;浏览器会自动转换成:HTTP://www.163.com/
2、获取IP,建立TCP连接
浏览器地址栏中输入"HTTP://www.xxx.com/"并提交之后,首先它会在DNS本地缓存表中查找,如果有则直接告诉IP地址。如果没有则要求网关DNS进行查找,如此下去,找到对应的IP后,则返回会给浏览器。
当获取IP之后,就开始与所请求的Tcp建立三次握手连接,连接建立后,就向服务器发出HTTP请求。
3、客户端浏览器向服务器发出HTTP请求
一旦建立了TCP连接,Web浏览器就会向Web服务器发送请求命令,接着以头信息的形式向Web服务器发送一些别的信息,之后浏览器发送了一空白行来通知服务器,它已经结束了该头信息的发送。
4、Web服务器应答,并向浏览器发送数据
客户机向服务器发出请求后,服务器会客户机回送应答,
HTTP/1.1 200 OK
应答的第一部分是协议的版本号和应答状态码,正如客户端会随同请求发送关于自身的信息一样,服务器也会随同应答向用户发送关于它自己的数据及被请求的文档。
Web服务器向浏览器发送头信息后,它会发送一个空白行来表示头信息的发送到此为结束,接着,它就以Content-Type应答头信息所描述的格式发送用户所请求的实际数据
5、Web服务器关闭TCP连接
一般情况下,一旦Web服务器向浏览器发送了请求数据,它就要关闭TCP连接,然后如果浏览器或者服务器在其头信息加入了这行代码
Connection:keep-alive
TCP连接在发送后将仍然保持打开状态,于是,浏览器可以继续通过相同的连接发送请求。保持连接节省了为每个请求建立新连接所需的时间,还节约了网络带宽。
Monday, December 7, 2009
SyncML 简介
SyncML是一种唯一行业通用的移动数据同步化协议,由SyncML initiative发行,是一种开放性协议。SyncML协议的目的是解决移动设备和网络之间的数据同步和设备管理问题。在SyncML之前,数据同步和设备管理是基于各种私有协议实现的,每种协议只能支持有限的设备和数据类型。各种协议间不能互通,这就限制了用户的数据访问、传输和移动性。
SyncML的主要目的有两方面:
* 可以通过任何移动设备将网络数据同步化。
* 移动设备中的数据也可以用任何网络数据同步化。
* SyncML协议特性:
* 可以在不同的网络上工作–包括有线网络和无线网络.
* 支持多种传输协议,包括HTTP,WSP,OBEX,SMTP,Pure TCP/IP.
* 支持通用的个人数据格式,如vCard,vCalendar和E-MAIL等.
* 考虑到移动设备的资源限制,对移动设备的存储空间进行了优化.
* 建立在internet协议和web技术上,是可执行而且有很好的协作性的.
* 协议最小功能就是赋予所有设备最常用的同步能力
Syncml的7种同步模式
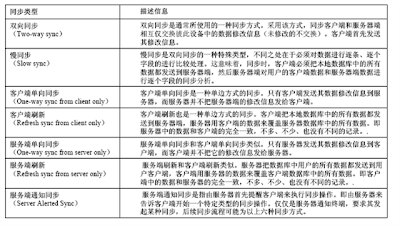
SyncML Protocol Architecture
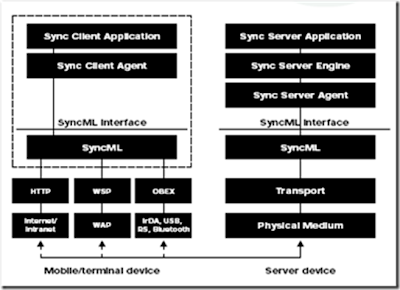
Friday, December 4, 2009
SELINUX从理解到动手配置
SELINUX从理解到动手配置
http://www.xxlinux.com/linux/article/network/security/20090531/16821.html
SELinux 可以为你的系统提供较棒的安全防护。 使用者能被分配预先定义好的角色,以便他们不能存取文件或者访问他们不拥有的程序。
Selinux的启用与关闭
编辑/etc/selinux/conf文件
SELINUX=enforcing(强制:违反了策略,你就无法继续操作下去)
Permissive(有效,但不强制:违反了策略的话它让你继续操作,但是把你的违反的内容记录下来)
Disabled(禁用)
禁用的另一种方式:在启动的时候,也可以通过传递参数selinux给内核来控制它
编辑/etc/grup.conf
title Red Hat Enterprise Linux Server (2.6.18-8.el5)
root (hd0,0)
kernel /vmlinuz-2.6.18-8.el5 ro root=LABEL=/ rhgb quiet selinux=0
initrd /initrd-2.6.18-8.el5.img
SELINUXTYPE=targeted
此参数可选项:targeted和strice。分别是targeted只控制关键网络服务,strice控制所有的服务
查询selinux的状态
[root@linuxas ~]# /usr/sbin/getenforce
Enforcing
[root@linuxas ~]# sestatus -bv
SELinux status: enabled
SELinuxfs mount: /selinux
Current mode: enforcing
Mode from config file: enforcing
Policy version: 21
Policy from config file: targeted
查看selinux加载的内核模块
[root@linuxas selinux]# semodule -l
amavis 1.1.0
ccs 1.0.0
clamav 1.1.0
dcc 1.1.0
evolution 1.1.0
iscsid 1.0.0
mozilla 1.1.0
mplayer 1.1.0
nagios 1.1.0
oddjob 1.0.1
pcscd 1.0.0
pyzor 1.1.0
razor 1.1.0
ricci 1.0.0
smartmon 1.1.0
查看selinux错误日志
[root@linuxas selinux]# sealert -a /var/log/audit/audit.log
SElinux的图形化管理工具
[root@linuxas selinux]# system-config-selinux
Selinux的基本操作
查看文件:ls –Z(--context)
[root@linuxas ~]# ls -Z
drwx------ root root root:object_r:user_home_t Desktop
-rw------- root root system_u:object_r:user_home_t anaconda-ks.cfg
-rw-r--r-- root root root:object_r:user_home_t install.log
-rw-r--r-- root root root:object_r:user_home_t install.log.syslog
[root@linuxas ~]# ls --context
drwx------ root root root:object_r:user_home_t Desktop
-rw------- root root system_u:object_r:user_home_t anaconda-ks.cfg
-rw-r--r-- root root root:object_r:user_home_t install.log
-rw-r--r-- root root root:object_r:user_home_t install.log.syslog
查看文件系统的扩展属性:getfattr
[root@linuxas ~]# getfattr -m. -d /etc/passwd
getfattr: Removing leading '/' from absolute path names
# file: etc/passwd
security.selinux="system_u:object_r:etc_t:s0�00"
查看的文件的 security.selinux 属性中储存了此文件的安全上下文, 所以上面例子中的上下文就是 system_ubject_r:etc_t 。
所有运行了SE Linux的ext2/3文件系统上都有 security.selinux 这个属性。
更改文件的扩展属性标签:chcon (不能在 /proc 文件系统上使用,就是说 /proc 文件系统不支持这种标记的改变。)
[root@linuxas test]# ls --context aa.txt
-rw-r--r-- root root root:object_r:user_home_t aa.txt
[root@linuxas test]# chcon -t etc_t aa.txt
[root@linuxas test]# ls --context aa.txt
-rw-r--r-- root root root:object_r:etc_t aa.txt
恢复原来的文件标签: restorecon
[root@linuxas test]# restorecon aa.txt
[root@linuxas test]# ls -Z aa.txt
-rw-r--r-- root root user_u:object_r:user_home_t aa.txt
显示当前用户的Selinux context
[root@linuxas ~]# id -Z
root:system_r:unconfined_t:SystemLow-SystemHigh
runcon 使用特定的context来执行指令
[root@linuxas ~]# runcon -t user_home_t cat /etc/passwd
root:system_r:user_home_t:SystemLow-SystemHigh is not a valid context
查看某种服务是否受到SELinux的保护
[root@linuxas ~]# getsebool -a (RHEL4:inactive受保护,active不受保护;RHEL5:off受保护,on不受保护)
Thursday, December 3, 2009
Eclipse插件开发小结
http://wjj-tt.spaces.live.com/blog/cns!91CCF857592F26C!106.entry
这两天看了一下Eclipse的插件开发,参考的书就是那本著名的Contributing to Eclipse。
把两点体会记录如下:
1、Extension和Extension-point
Extension 和Extension-point是相互关联的,由Extension-point定义插件具备的扩展点并且定义了如何使用这些扩展点(由Schema中 定义),而Extension则是根据扩展点中定义的Schema来描述扩展的内容。Extension定义的是对已有插件进行的扩展,其中point是 扩展源的id。对于不同的扩展源,Extension的元素定义各不相同。Extension-piont定义的是本插件向外提供的扩展功能,利用 Schema来定义Extension。
【学习心得】JFace和SWT有什么区别呢?
JFace:插件的用户界面框架
我们已经见到工作台定义扩展点来为使插件向平台添加用户界面功能。许多这些扩展点,特别是向导扩展,是通过使用 org.eclipse.jface.* 包中的类来实现的。有什么区别?
JFace 是一个用户界面工具箱,它提供很难实现的、用于开发用户界面功能部件的 helper 类。JFace 在原始的窗口小部件系统的级别之上运行。它提供用于处理常见的用户界面编程任务的类:
查看器负责处理填充、排序、过滤和更新窗口小部件等最辛苦的工作。
操作和添加项介绍用于定义用户操作的语义,并指定在何处提供它们。
图像和字体注册表提供用于处理用户界面资源的常见模式。
对话框和向导定义用于构建与用户进行复杂交互的框架。
JFace 使您可以专注于实现特定插件的功能,而不必花费精力来处理底层窗口小部件系统或者解决几乎在任何用户界面应用程序中都很常见的问题。
JFace 和工作台
何处是 JFace 结束而工作台开始的位置?有时候界线并不是这样明显。通常,JFace API(来自于包 org.eclipse.jface.*)独立于工作台扩展点和 API。可以想象,根本不必使用任何工作台代码就可以编写 JFace 程序。
工作台使用 JFace,但是又试图尽可能减少依赖项。例如,工作台部件模型(IWorkbenchPart)被设计为独立于 JFace。我们很早就知道可以直接使用 SWT 窗口小部件来实现视图和编辑器,而不必使用任何 JFace 类。工作台尽可能保持“JFace 中立”,允许程序员使用他们觉得有用的 JFace 的部件。实际上,在工作台的大多数实现中都使用了 JFace,并且在 API 定义中引用了 JFace 类型。(例如,IMenuManager、IToolBarManager 和 IStatusLineManager 的 JFace 接口显示为工作台 IActionBar 方法中的类型。)
JFace 和 SWT
SWT 和 JFace 之间的界线是很明显的。SWT 根本不依赖于任何 JFace 或平台代码。许多 SWT 示例说明可以如何构建独立的应用程序。
JFace 用来在 SWT 库顶部提供常见的应用程序用户界面功能。JFace 并不试图“隐藏”SWT 或者替换它的功能。它提供一些类和接口,以处理与使用 SWT 来对动态用户界面编程相关联的许多常见任务。
通过了解查看器及它们与 SWT 窗口小部件之间的关系,就可以更清楚地了解 JFace 和 SWT 之间的关系。
Java是一种强大的编程语言。但强大就意味复杂,尤其是和Java相关的名词就象天上的星星一样,数都数不过来。在本文中就涉及到两个比较常用的名词 SWT和JFace。在标题中将SWT和JFace放到一起,并不是说SWT和JFace是一个意思,而是说它们的关系非常紧密。
基于Java的图形库最主要的有三种,它们分别是Swing、AWT和SWT。其中前两个是Sun随JDK一起发布的,而SWT则是由IBM领导的开源项目(现在已经脱离IBM了)Eclipse的一个子项目。SWT的执行效率非常高。这是由于SWT的底层是由C编写的。由于SWT通过C直接调用系统层的 GUI API。因此,使用SWT编写GUI程序,在外观上就和使用C++、Delphi(在Windows下)编写的程序完全一样。它的这一点和AWT类似。 AWT在底层也是使用C直接调用系统层的GUI API。但它们是有区别的,最大的区别可能就是一个是Sun提供的,一个是Eclipse自带的。这就意味着如果使用AWT,只要机器上安装了JDK或 JRE,发布软件时无需带其它的库。而如何使用SWT,在发布时必须要自带上SWT的*.dll(Windows版)或*.so(Linux/Unix 版)文件以及相关的*.jar包。还有就是它们所提供的图形接口有一些差异。SWT可能更丰富一些,我们可以看看Eclipse的界面就知道了。但随着 Sun对AWT库的不断更新,AWT的图形表现能力也在不断地提高。
虽然SWT很强大,但它比较底层。也就是说它的一些功能在使用上还比较低级,不太符合面向对象的特征。因此,在SWT的基础上又开发了JFace。JFace在SWT上进行了一定的扩展。因此,也可说JFace是基于 SWT的,就象在VC中使用MFC来包装Win32 API一样。
Wednesday, December 2, 2009
What is Spring?
http://onjava.com/onjava/2005/10/05/what-is-spring.html
Spring is a lightweight container, with wrappers that make it easy to use many different services and frameworks. Lightweight containers accept any JavaBean, instead of specific types of components.
"Spring is more than just a 'lightweight container,'" says Justin Gehtland. "It allows Java developers who are building J2EE apps to get to the heart of their real domain problems and stop spending so much time on the minutiae of providing services to their domain." Gehtland and Bruce Tate are coauthors of Spring: A Developer's Notebook, a no-nonsense book that will get you up to speed quickly on the new Spring open source framework. Spring: A Developer's Notebook includes examples and practical applications that demonstrate exactly how to use Spring, in ten chapters of code-intensive labs.
Today we'll feature the first of two chapters from the book, in our two-part series dubbed "What Is Spring." The series will help you understand how you can use Spring to produce simple, clean, and effective applications.
In this week's excerpt of Chapter 1, "Getting Started," authors Tate and Gehtland take a simple application and show you how to automate it and enable it for Spring. (In part 2 next week, the authors will cover how Spring can help in development of simple, clean, web-based UI's--excerpted from Chapter 2, "Building a User Interface.")
Introduction to Eclipse Plugin Development
From : http://www.eclipsepluginsite.com/index.html
Eclipse Plugin Development TUTORIAL
Eclipse Plugin Development Tutorial website to teach you how to develop eclipse plugins using simple examples to a complex eclipse rcp over time. This chapter will give you a detailed insight into Eclipse Architecture and we will develop a simple but fully functional eclipse plug-in so as to give you a quick start with eclipse plug-in development.
Overview
Eclipse isn’t a huge single java program, but rather a small program which provides the functionality of typical loader called plug-in loader. Eclipse (plug-in loader) is surrounded by hundreds and thousands of plug-ins. Plug-in is nothing but another java program which extends the functionality of Eclipse in some way. Each eclipse plug-in can either consume services provided by other plug-in or can extend its functionality to be consumed by other plug-ins. These plug-in are dynamically loaded by eclipse at run time on demand basis.
An Open Platform
Eclipse is an open platform. It is designed to be easily and infinitely extensible by third parties. At the core is the eclipse SDK, we can build various products/tools around this SDK. These products or tools can further be extended by other products/tools and so on. For example, we can extend simple text editor to create xml editor. Eclipse architecture is truly amazing when it comes to extensibility. This extensibility is achieved by creating these products/tools in form of plug-ins.
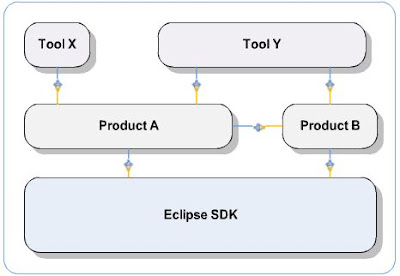
Figure 1-1
Inside the Eclipse SDK
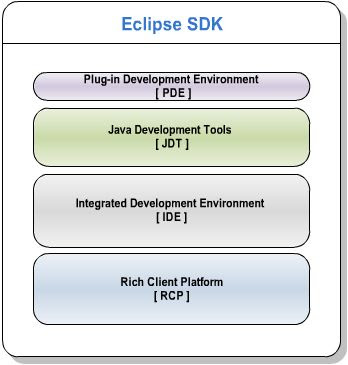
Figure 1-2
RCP: On the bottom is RCP which provides the architecture and framework to build any rich client application.
IDE: It is a tools platform and a rich client application itself. We can build various form of tooling by using IDE for example Database tooling.
JDT: It is a complete java IDE and a platform in itself.
PDE: It provides all tools necessary to develop plug-ins and RCP applications. This is what we will concentrate on the course of this tutorial.
Plug-ins everywhere
All the layers in eclipse SDK are made up of plug-ins. If you see all the way, you will notice that everything is a plug-in in eclipse sdk.
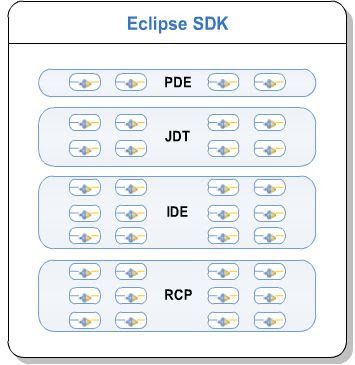
Figure 1-3
Plug-in Architecture
A plugin is a small unit of Eclipse Platform that can be developed separately. It must be noted that all of the functionality of eclipse is located in different plugins (except for the kernel)
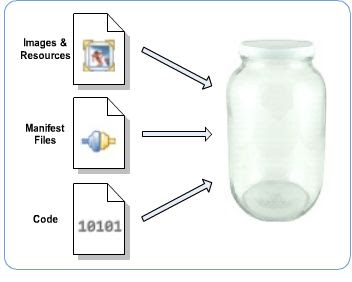
A plug-in can be delivered as a jar file. A plug-in is self-contained bundle in a sense that it contains the code and resources that it needs to run for ex: code, image files, resource bundles etc. A plug-in is self describing - when I say it is self describing it means that it describes who it is and what it contributes to the world. It also declares what it requires from the world.
A Mechanism For Extensibility
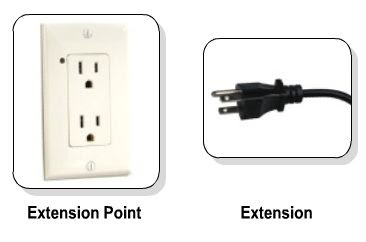
Figure 1-7
We all know that eclipse is extensible. In order to achieve this extensibility eclipse uses the concept of extension points and extension. Think of extension point as Electric socket and think of extension as a plug. Plug-in A exposes a extension point (electric socket) which Plug-in B extends by providing an extension (an electric plug). Plug-in A knows nothing about plug-in B. If we think about it this is very similar to concept of Inheritance – we extend functionality of base class to provide more specific implementation. Think of Plug-in A as a text editor and Plug-in B as xml editor. Text editor declares extension point thereby declaring to the world that it is open for extension and xml editor extends text editor by using its extension point to customize it in its own way. It is important to understand that each extension point essentially declares
a contract. An extension point provider only accepts those extensions which abide by the contract.
These extension points and extensions are declared in plugin.xml (discussed earlier). The runtime is able to wire extensions and extension points and form a registry using markup alone.
Plug-in Example
Now that we have covered good amount of architecture basics, its time to get our hands dirty with some actual plug-in coding. The process for creating a plug-in is best demonstrated by implementing a plug-in on which discussion and examples can be based. Here we will take a step-by-step approach to create a simple but fully operational plug-in. This example will try to give you an feel of eclipse plug-in development. However, don’t try to grab all the details at this point. It is fine if you are not able to understand much of the details in this example. Rest of the tutorial will cover all the topics in great depth.
We will build a simple Resource Manager plug-in. Features of this plug-in are as follows:
- Ability to add/remove resources (essentially files in workspace) to resource manager.
- Display list of resources which were added to resource manager.
- Ability to open up associated editor whenever resource is clicked.


Tuesday, December 1, 2009
Monday, November 30, 2009
Friday, November 20, 2009
爱心女摄影师的哀婉遗作
对 于一名野生动物摄影师来说,图片中这些小家伙绝不是最令人感到威胁的猫科动物。它们长着松柔滑的皮毛、明亮而伶俐的眼睛以及柔软的爪子,是如假包换的 猫。这些令人陶醉的图片是由野生动物摄影师简·伯顿拍摄的。由于可怕的癌症,伯顿于2007年不幸去世,这些图片成为她摄影才能和忍耐力的一个令人心酸的 最后证明。
多年来,伯顿共与丈夫基姆·泰勒以及两个儿子收养了60多只猫,他们将这些小家伙安置在自己位于萨里的家。凭借令人难于置信的细节捕捉能力和强大的忍耐力 ——为了记录下心目中的完美时刻,伯顿有时会苦等4个小时——以及在与癌症抗争时表现出的勇气,伯顿为自己心爱的动物朋友——猫、松鼠和兔子——拍摄了一 幅幅精彩的照片。从某种程度上说,为宠物拍照已成为消除因危机四伏的野外拍摄产生的精神紧张的一剂良药。
文中这些可爱的照片将首次刊登在《猫咪生活》上,《猫咪生活》是伯顿去世后出版的第一部摄影作品集。伯顿是1960年离开英国前往西非的早期女性野生动物 摄影师,该摄影领域的先驱;当时的泰勒是一名农业顾问,正是他带着这些勇敢的女性来到尼日利亚。在多年的野外拍摄过程中,他们的足迹遍布西非,空余时间还 制作了几部纪录片,其中包括为独立电视台(ITV)拍摄的《蝙蝠洞》以及为英国广播公司(BBC)拍摄的《加勒比海摄影之旅》。
尽管在世界范围内从事野生动物拍摄工作,但据泰勒透露,用镜头捕捉猫的本性和特征才是妻子最出众的才能。他说:“在工作室进行拍摄时,猫是非常不合作的拍摄对象,但简却做到了很多摄影师无法做到的事情。作为一项最为出众的才能,她知道应在何时准确按下快门。”
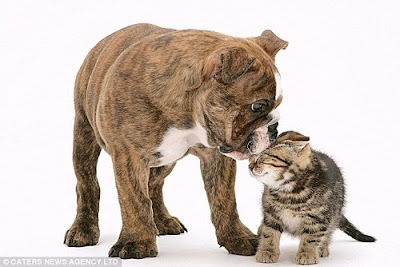
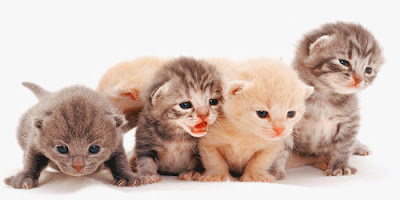
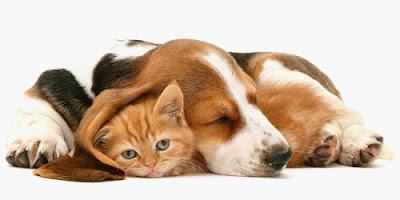
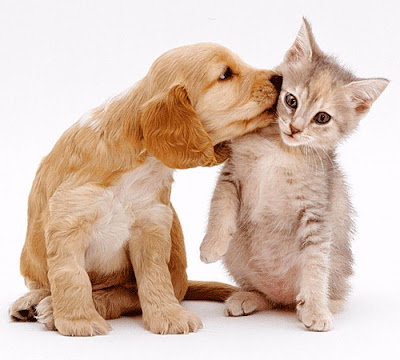
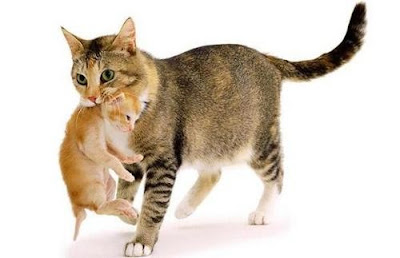
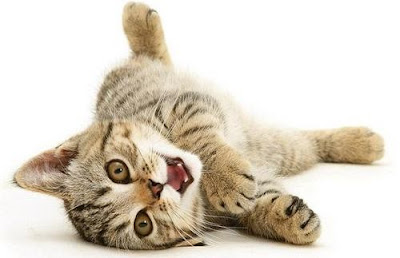
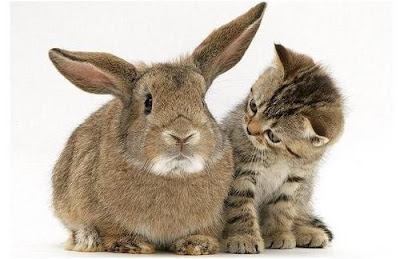
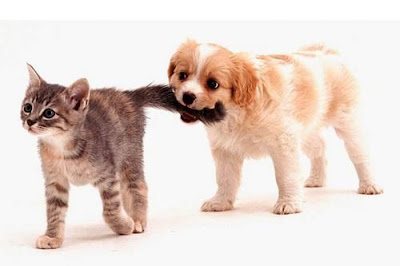
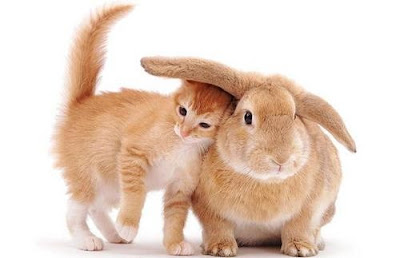
Thursday, November 19, 2009
希腊罗马神话和《圣经》中的英语典故【22】
22.a wolf in sheep's clothing批着羊皮的狼;貌善心恶的人
耶稣在加利利一带传道布教,收了很多信徒。有一天,他对门徒说:"Beware of false prophets,which come to you in sheep's clothing,but inwardly they are ravening wolves"
eg:Mrs.Martin trusted the lawyer until she realized that he was a wolf in sheep's clothing
One who teaches morality and practises immorality is a wolf in lamb's skine
23.separate the sheep from the goats区别好坏,分清良莠
《新约。马太福音》记述:“And before him shall be gathered all nations:and he shall separate them one from another,as a shepherd divideth his sheep from the goats”
由于《圣经》的影响,sheep和goat在英语中的形象截然不同,前者比喻好人,后者比喻坏人。英语中有关goat的成语,大多贬义。如:to play the goat=play the fool(瞎胡闹);to get sb's goat(触动肝火) ;等等。《圣经》说牧羊人要分辨绵羊和山羊,“把绵羊安置右边,山羊左边”。据说野山羊常混进羊群里,引诱绵羊,故牧养人必须把它们区分开来,以免混淆。
由此,人们用to separate the sheep from the goats这个成语,来比喻to separate the good from the wicked; to divide good or useful people from bad or useless
eg:We'll go through the list of members,and separate the sheep from the goats
Have faith in me,please.I can separate the sheep from the goats
希腊罗马神话和《圣经》中的英语典故【21】
21.Cast pearls before swine对牛弹琴;白费好意
To Cast pearls before swine的意思是“珍珠头在猪猡前面”。
swine是个旧词,书面词,即今为pigs,不过swine单复同行,本句为复数。
这个成语源自《新约。马太福音》第7章:“Give not that which is holy unto the dogs,neither cast ye your pearls before swine,lest they trample them under their feet,and turn again and rend you”.由于to cast pearls efore swine,比喻确切,在后世不断引用中而成为一个国际性成语,常用来表示to offer sth valuable or beautiful to those who can't appreciate it;to give what is precious to those who are unable to understand its value等意思,含有轻蔑嘲笑色彩。按其字面意义,这个成语与汉语成语“明珠按投”相似,但是寓意不同,基本上不对应;按一比喻意义,它相当于“对牛弹 琴”,“向驴说经”“一番好意给狗吃”“狗咬吕洞宾,不识好人心”等。
She read them Shakespeare,but it was casting pearls before swine
I won't waste good advice on John any more because he never listens to it.I won't cast pearls before swine.
...and when I let the upper floor to Cap'en Cuttle,oh i do a thankless thing,and cast pearls before swine.
希腊罗马神话和《圣经》中的英语典故【20】
20.The Salt of the Earth社会中坚;民族精华;优秀份子
The Salt of the Earth这个成语,字面意思“世上的盐”
盐是饮食中不可缺少的调味品,人体若缺盐,健康就会受到影响,出现种种疾病。盐还有杀菌、解毒、消炎、除污等多种功用,它既是“百药之王”,又是工业之母,确是值得珍视的东西。在许多民族的习俗汇总,盐被当作敬客的高贵礼品。
The Salt of the Earth一词出自《圣经》,据《新约。马太福音》(Matthew)第5长记载:耶稣对他的门徒说:"Ye are the salt of the earth:but if the salt have lost his savor,wherewith shall it be salted? " 在这里,salt用于转义,表示flavor;of the earth即of the world。这是耶稣登山垂训论"福",所讲福音结尾的话,他把门徒比做“世上的盐”,这是极高的称赞。这句话在后世不断引用变成了一个典故性成语,转义 为the most valuable members of sociey;the finest type of humanity;a person or a group of people having the best character 之意
eg: He does a lot of good jobs and is considered to be the salt of the world.
You all are the salt of the earth.Our hope is placed on you.
希腊罗马神话和《圣经》中的英语典故【19】
19.Not an iota of没有一点点,丝毫也不
iota是希腊字母表中第9个字母“I”的名称。not an iota of 出自《新约。马太福音》第5章:“律法的一点一画都不能废去,都要成全。”因为iota是希腊字母表中最小的一个字母,它有时可以写作一短横置于其他字母 之上;遗漏这一点点对发音并无什么影响,只按规则不能减少而已。《福音书》所说的律法,系指“摩西律”,意即无论何人都不允许随便废去这戒律哪怕是最小的 一条,甚至其中的一个字母,一个小小短横也不得更动或遗漏。
由此,在语言中遗留下来这个成语,转义表示not a bit of ;not one jot or little;not at all等意思。iota在这里,相当于汉语“小不点儿”的意思。
Eg:Science deals with things in a practical way.Science means honest,solid knowledge,allowing not an iota of falsehood,and it involves herculean efforts and gruelling toil.
There is not an iota of truth in the story.
希腊罗马神话和《圣经》中的英语典故【18】
18.The writing/Finger on the Wall不详之兆;大祸临头
这个成语的字面意思是“墙上的文字(或手指)”,而实际含义是a sign or warning of impending disaster(迫在眉睫的凶兆);a sign that sth bad will happen; a feeling that ones number is up;等等。其语言外壳与内涵是怎样联系起来的呢?还是出自《圣经》
据《旧约。但以理书》(Daniel)第5章记述:有一次古巴比伦(Babylonian)的国王伯沙撒(Belshazar)正在宫殿里设宴 纵饮时,突然,不知从哪里出现了一个神秘的手指,当者国王的面,在王宫与灯台相对的粉墙上写西了四个奇怪的单词:MENE(弥尼)、MENE(弥尼)、 TEKEL(提客勒)、UPHARSING (乌法珥新)。国王张皇失措,惊恐万分,谁也不懂墙上所写的字是什么意思。后来叫来了被虏的犹太预言家但以理,才明白了这几个字的意思就是大难临头。他 说:“弥尼就是上帝已经数算你国的年日到此为完毕;提客勒就是你被称在天平里显出你的亏欠;乌法珥新就是你的国分裂,归与玛代人和波斯人。”果然,当夜伯 沙撒被杀,又62岁的玛代人大利乌取而代之。
依次,“墙上的文字(或手指)”就表示身死国亡的凶兆。英语中这个成语有几种表达方式:the writing/handwriting on the wall或a finger on the wall,通常与be,like等系动词连用;有时写成see/read the writing on he wall的句型,表示提出警告,such as :Don't you see the writing on the wall, 有时候也可省略on the wall,只说Don't you see the writing?意思也是一样的。
eg:This inexplicable incident seemed,like the Babylonian finger on the wall,to be spelling out the letter of my judgement...
In this house of his there was writing on every wall.His business-like temperament protested against a mysterious warning that she was not made for him.
John's emplyer had less and less work for him;John could read the writing on the wall.
The writing on the wall is clear:if man behaves like an animal and allow hs population to increase while each nation steadily increases he coplexity and range of its environment,nature will take her course and the law of the Jungle will prevail.
When Bill's team lost four games in a row,he saw the handwriting on the wall.
希腊罗马神话和《圣经》中的英语典故【17】
17.The Apple of Ones' Eye
The Apple of Ones' Eye的字面意思是“某人眼里的苹果”,在这里,apple指的是the pupil(瞳孔,眼珠),大概因眼珠圆的象苹果之故。瞳孔是眼睛最重要的部 分,失去瞳孔,光线就无法通过虹膜中心的圆孔进入眼内而变成了瞎子。所以,这个成语常用来比喻象爱护眼珠一样爱护某个最心爱的人或珍贵的东西,即表示a cherished person or object;sth extrmely precious to one; sb dearly loved等意
这个成语来字《旧约。申命记》(Deuteronomy)第32章“耶和华遇见他在旷野荒凉、野兽吼叫之地,就环绕他,看顾他,保护他如同保护眼里 的瞳人。”在圣经其他地方也有类似的话。英文版《旧约。诗篇》(Psalm)第17章有这样的句子:"Keep me as the apple of the eye,hide me under the shadow of the wings"
成语the apple of one's eye是固定结构,不得写成the apple of the eye of…的形式;在搭配上,它常与动词be,keep,care for等连用。按其想象意义,它与汉语成语“掌上明珠”颇相似,但其比喻的对象较汉语“掌 珠”更广,因“掌珠”通常指心爱的女儿,而不能用与其他场合。
eg:Little Mary is the apple of her father's eye
Mind the reputation of your school as you care for the apple of your eye.
希腊罗马神话和《圣经》中的英语典故【16】
16.Sell One's Birthright for a Mess of Pottages因小失大;见利弃义
Sell One's Birthright for a Mess of Pottages直译是:“为了一碗红豆汤而出卖了长子继承权”。
《旧约.创世纪》第25章记述了这样一个故事传说:犹太族长以撒的妻子利百加怀孕期间,感觉到2个胎儿在她腹内互相踢打,就去问耶和华,耶和华对她说:“两国在你腹内,两族要从你身上出来,这族必强于那族,将来大的要服小的。”
后来,利百加果然生下一对孪生兄弟,哥哥叫以扫,弟弟叫雅各。两兄弟长大后,以扫好动,常外出打猎;雅各则常在家里帮助料理家务。有一天,以扫打猎回 来,又饥又渴,看见弟弟雅各在熬豆汤,就对他说:“我饿极了,给我喝点红豆汤吧!”雅各说:“你要喝汤,就把你的长子权卖给我。”以扫说:“你都要饿死 了,要这长子权有什么用呢?”于是,他便按雅各的要求,对天起誓,把长子权卖给雅各,换来饼和红豆汤。以扫吃饱喝足后,起身走了。他哪里想到,为了这碗红 豆汤,他的后裔便注定要服事雅各的后裔。
由此,人们用to sell one's birthright for a mess of pottage短语,来比喻to exchange something of lasting value for something that is of value for a short time only;to suffer a big loss for a little gain.这个成语常缩略为for a mess of pottage的形式。有时也可用to sell one's birthrights.
eg:It was argued that joining the Common Market...would be giving away her national rights and advantages for a mess of pottage.
There are many,many people who are willing to prostitute their intelligence for a mess of pottage.
希腊罗马神话和《圣经》中的英语典故【15】
15.Adam's Apple喉结
亚当是圣经中人类的始祖,而苹果的历史比人类的历史还悠久。在世界各文明古国的民间故事和神话传说中,苹果都是受人喜爱的一种果实。英语中有个谚 语:An apple a day keeps the doctor away.但据圣经故事上说,苹果也给人类带来了麻烦,男人的喉结就是因吃苹果引起的。
《旧约.创世纪》第3章讲到人类的起源,传说上帝创造人类的始祖亚当和夏娃,在东方的伊甸(Eden)建立了一个园子给他们居住。伊甸园里生长着悦人 眼目的各种树木,树上长着各种各样的果实。上帝吩咐亚当说:你可以随意吃园中的各种果子,只是不能吃那棵分别善恶树上的果实,吃了必定要死。这种“禁果” 就是apple。后来,亚当的配偶夏娃听信蛇的诱惑,不顾神谕,吃了善恶树上的禁果,还把这果子给它丈夫吃。亚当因心怀恐惧,吃时仓促,有一片果肉哽在吼 中,不上不下,留下个结块,就叫“亚当的苹果”两人吃了这果子就心明眼亮,能知善恶美丑。但是由于他们违背了上帝的告戒而被逐出伊甸园。从此,亚当就永远 在脖子前端留下“喉结”,作为偷吃禁果的“罪证”。上帝还惩罚亚当,“必汗流满面才能糊口”
不过也说一说是正当亚当吃的时候,上帝来了,所以亚当急忙吞下去,不料哽在喉咙间了。
eg:Your Adam's apple isn't apparent.
Adam's apple can be more clearly seen on men than women's throats.
希腊罗马神话和《圣经》中的英语典故【14】
14.Bone of The Bone and Flesh of the Flesh
Bone of The Bone and Flesh of the Flesh直译"骨中之骨,肉中之肉",出自<圣经>中关于上帝造人的神话.
据<旧约·创世纪>第2章叙述:太初之际,混沌未开,耶和华上帝开天辟地.第一天耶和华创造了白天和夜晚;第二天创造了天空和风云;第三 天创造了高山峻岭.平原河流,以及富饶的土地和芳香的花果;第四天他又创造了太阳.月亮和星辰,确定年岁.季节.月份和日期;第五天他创造了各种形状和大 小的鱼类和飞禽;第六天他才创造了各种陆上动物,然后他按照自己的形象用地上的尘土造出一个男人,名叫亚当(Adam),这就是神话中人类的始祖.后来, 耶和华见押当独居无伴侣帮助他,于是,趁亚当沉睡的时候,从他身上取下一根肋骨造成了一个女人叫夏娃(Eve),领到他面前,亚当说:"This is bone of my bone and flesh of my flesh"(这是我骨中之骨,肉中之肉)。从此两人结为夫妻
Bone of The Bone and Flesh of the Flesh常用来比喻血缘上的亲属关系或思想上的团结一致,即as close as flesh and blood;to be inseperately linked to each other等的意思。
eg:Our army is bone of the bone and flesh of the flesh of the people.
The I.W.W was bone of the bone and flesh of the flesh of the floating workers.(W.Foster:Pages from a Worker's Life.)
希腊罗马神话和《圣经》中的英语典故【13】
13.Cut the Gordian Knot
Cut the Gordian Knot直译“斩断戈耳迪之结”,源自上篇的同一典故。
佛律基亚(Phrygia)的国王戈耳迪,用乱结把轭系在他原来使用过的马车的辕上,其结牢固难解,神谕凡能解开此结者,便是亚洲之君主。好几个世纪 过去了,没有人能解开这个结。公元前3世纪时,古希腊罗马的马其顿国王亚历山大大帝(Alexander the Great,公元前356-323),在成为希腊各城邦的霸主后,大举远征东方。公元前334年,他率领进入小亚细亚,经过佛律基亚时,看到这辆马车。有 人把往年的神谕告诉他,他也无法解开这个结。为了鼓舞士气,亚历山大拔出利剑一挥,斩断了这个复杂的乱结,并说:“我就是这样解开的”因此,to cut the Gordian knot 就是意味着to solve a complicated difficulty by quick and drastic action;to end a difficulty by using a vigorous or violent method;to solve a problem by force.按其形象意义,这个成语与汉语成语“快刀斩乱麻”,“大刀阔斧,果断处置”十分相似。
eg:They have decided to cut the Gordian knot to wipe out the enemy at a blow.
Jean is afraid of everything,How can she cut the Gordian knot in her work?
希腊罗马神话和《圣经》中的英语典故【12】
12.A Gordian Knot难解的结;难题;难点
A Gordian Knot直译“戈耳迪之结”。
戈耳迪(Gordius)是小亚细亚佛律基亚(Phrygia)的国王,传说他原先是个贫苦的农民。一天,他在耕地的时候,有只神鹰从天而且降,落在 他马车的轭上,久不飞走。戈耳迪就赶着马车进城去请求神示。其时,佛律基亚的老王突然去世,一国无主,上下动乱不安,于是人们请求神示由谁来做国王。神示 说:“在通向宙斯神庙的大陆上,你们遇到的第一个乘马车者就是新王。”恰好这时戈耳迪正乘着牛车前往宙斯的神庙,人们看见巍然屹立在车轭上的神鹰,认为这 是掌握政权的象征,就一致拥戴戈耳迪为国王。戈耳迪当了国王后,就把那辆象征命运的马车献给宙斯,放置在婶庙中。他用绳索打了个非常复杂的死结,把车轭牢 牢得系在车辕上,谁也无法解开。
由此,人们常用a Gordian knot比喻a knot difficult or impossibe to unite;the difficult problem or task.
eg:We must try to solve the problem even if it is really a Gordian knot.
The knot which you thought a Gordian one will untie it before you.
希腊罗马神话和《圣经》中的英语典故【10】
11.A Procrustean Bed
A Procrustean Bed直译是“普洛克路斯贰斯的床”,源自古希腊神话的典故。
在雅典国家奠基者(Theseus)的传说中,从墨加拉到雅典途中有个非常残暴的强盗,叫达玛斯贰斯,绰号普洛克路斯贰斯。希腊问 Procrustes的意思是“拉长者”、“暴虐者”。据公元前1世纪古希腊历史学家狄奥多(Diodoros,约公元前80-前29年)所编《历史丛 书》记述:普洛克路斯贰斯开设黑店,拦截过路行人。他特意设置了2张铁床,一长一短,强迫旅店躺在铁床上,身矮者睡长床,强拉其躯体使与床齐;身高者睡短 床,他用利斧把旅客伸出来的腿脚截短。由于他这种特殊的残暴方式,人称之为“铁床匪”。后来,希腊著名英雄提修斯在前往雅典寻父途中,遇上了“铁床匪”, 击败了这个拦路大盗。提修斯以其人之道还治其人之身,强令身体魁梧的普洛克路斯贰斯躺在短床上,一刀砍掉“铁床匪”伸出床外的下半肢,除了这一祸害。
由此,在英语中遗留下来a Procrustean bed这个成语,亦做the Procrustes' bed或the bed of Procrustes,常用以表示an arrangement or plan that produces uniformity by violent and arbitrary measures之意。按其形象意义,这个成语与汉语成语“削足适履”、“截趾穿鞋”颇相同;也类似俗语“使穿小鞋”、“强求一律”的说法
eg:I didn't put forth the plan as a Procrustean bed,to which exact conformity is to be indispensable.
Don't stretch the facts to fit the Procrustean bed.
希腊罗马神话和《圣经》中的英语典故【9】
9.Under the Rose秘密地;私下得;暗中
Under the rose直译"在玫瑰花底下",而实际上却表示in secret; privately confidentially的意义,语言外壳与内涵,似乎风马牛不相及.它源自古罗马的神话故事和欧洲的风尚.
罗马神话中的小爱神丘比特(Cupid),也称希腊神话里的厄洛斯(Eros),在文艺作品中以背上长着双翼的小男孩的形象出现,常携带弓箭在天空中 遨游,谁中了他的金箭就会产生爱情.丘比特是战神玛斯(Mars)和爱与美之神维纳斯(venus)所生的儿子.维纳斯,也就是希腊神话里的阿芙罗狄蒂 (Aphrodite),传说她是从大海的泡沫里生出来,以美丽著称,从宙斯到奥林匹帕斯的诸神都为起美貌姿容所倾倒.有关她的恋爱传说很多,欧洲很多文 艺作品常用维纳斯做题材.小爱神丘比特为了维护其母的声誉,给沉默之神哈伯克拉底(Harpocrates)送了一束玫瑰花,请他守口如瓶不要把维纳斯的 风流韵事传播出去.哈伯克拉底受了玫瑰花就缄默不语了,成为名副其实的"沉默之神"
古罗马人对维纳斯非常尊崇,不仅奉为掌管人类的爱情.婚姻.生育的爱与美的神,而且尊为丰收女神.园艺女神.罗马的统治者恺撒大帝甚至追搠维纳斯是罗 马人的祖先.由于上述神话传说,古罗马人把玫瑰花当作沉没或严守秘密的象征,并在日常生活中相尚成风.人们去串门做客,当看到主人家的桌子上方画有玫瑰, 客人就了解在这桌上所谈的一切行为均不应外传.于是在语言中产生了Sub rosa在玫瑰花底下这个拉丁成语. 据<牛津英语词典>解释,英语under the rose系源自德语unter der Rosen. 古代德国的宴会厅.会议室以及旅店的餐室,在天花板上常画有或雕刻着玫瑰花,用来提醒在场者要守口如瓶,严守秘密,不要把玫瑰花底下的言行透露出去.这个 流行于15至17世纪的德语成语反映了这种习俗.
罗马帝国全盛时,其势力几乎席卷了整个欧洲,罗马某些文化风尚也随着他的军事力量渗透到欧洲各国.因此,以玫瑰花象征沉默的习俗,并不限于德国..
under the rose 是个状语性成语,在句中修饰动词,其含义因所修饰的动词的不同而略有不同.如:born under the rose"私生的""非婚生的";do under the rose"暗中进行"
eg:The senator told me under the rose that there is to be a chance in the cabinet.
The matter was finally settled under the rose.
Do what you like undeer the rose,but don't give a sign of what you're about...
希腊罗马神话和《圣经》中的英语典故【8】
8.Win/Gain Laurels获得荣誉;赢得声望
Look to One's Laurels爱惜名声;保持记录
Rest on One's Laurels坐享清福;光吃老本
Laurel(月桂树)是一种可供观赏的常绿乔木,树叶互生,披针形或者长椭圆形,光滑发亮;花带黄色,伞形花序.laurels指用月桂树叶编成 的"桂冠".古代希腊人和罗马人用月桂树的树叶编成冠冕,献给杰出的诗人或体育竞技的优胜者,作为奖赏,以表尊崇.这种风尚渐渐传遍整个欧洲,于是 laurels代表victory,success和distincion.
欧洲人这种习俗源远流长,可上朔到古希腊神话.相传河神珀纳斯(Peneus)的女儿达佛涅(Daphne)长的风姿卓约,艳丽非凡.太阳神阿波罗为 她的美所倾倒,热烈追求她,但达佛涅自有所爱,总是逃避权利很大的太阳神的追求.一天,他俩在河边相遇,达佛涅一见阿波罗,拔腿就跑,阿波罗在后边穷追不 舍,达佛涅跑得疲乏不堪,情急之下只好请她父亲把她变成一株月桂树.阿波罗非常感伤,无限深情地表示:"愿你的枝叶四季长青,装饰我的头,装饰我的琴,让 你成为最高荣誉的象征".他小心得将这株月桂树移植到自己神庙旁边,朝夕相处,并取其枝叶遍成花冠戴在头上,以表示对达佛涅的倾慕和怀念.
因此,古希腊人把月桂树看做是阿波罗的神木,称为"阿波罗的月桂树"(The Laurel of Apollo).起先,他们用月桂枝叶编成冠冕,授予在祭祀太阳神的节目赛跑中的优胜者.后来在奥林匹亚(Olympia)举行的体育竞技中,他们用桂冠 赠给竞技的优胜者.从此世代相传,后世欧洲人以"桂冠"作为光荣的称号.
由于阿波罗是主管光明.青春.音乐和诗歌之神,欧洲人又把源自"阿波罗的月桂树"的桂冠,献给最有才华的诗人,称"桂冠诗人".第一位著名的"桂冠诗 人"就是欧洲文艺复兴时期人文主义的先驱者.意大利诗人彼特拉克(Francesco Petrarch,1304-1374).他的代表作<抒情诗集>,全部为14行诗体,系诗人献给他心中的女神劳拉的情诗(彼特拉克喜欢了劳 拉一辈子,但是劳拉从来都不知道),抒发他对恋人的爱情,描写大自然的景色,渴望祖国的统一.这部被称为西方"诗三百'的诗集,虽不能与我国古代< 诗经>相提并论,但不失为世界文学的瑰宝.
中古时代英国的大学,也曾授予过"桂冠诗人"的称好,但是这只是一种荣誉称号,而非目前含义的类似职务,学衔的专用名称.
作为专名的"桂冠诗人"(The Poet Laureate,也称The Laureate),系英国王室赐予御用诗人的专用称号,从17世纪英皇詹姆士一世(James I,1566-1625)开始,延续到现在,已历三个世纪了.凡获得"桂冠诗人"称号者,可领取宫廷津贴,每遇到王室喜庆或官方盛典时,都要写作应景诗以 点缀和宣扬喜庆事件,歌功颂德,粉饰升平.17世纪,在英国被封为第一位"桂冠诗人"的是约翰·德莱顿(John Dryden,1631-1700),他一生为贵族写作,美化君主制度,不过他创造的"英语偶句诗体",成为英国诗歌的主要形式之一.从1670到 1972这三百年间,英国王室相继封了17位"桂冠诗人"年限最长的是19实际的浪漫诗人阿弗里德·丁尼生(Alfred Tennyson,1809-1892),他从1850年获得这个称号一直到逝世,长达42年,算是"终身桂冠诗人"了.英国最近的"桂冠诗人"是约翰· 本杰明(John Benjamin).其实,所谓"桂冠诗人"大部分是徒具虚名的,在英国文学史上享有盛名者极少;就象中国封建时代的"钦点状元",从公元960到 1904(清关绪30年最后一届科举止)近1000年,历代状元341名,在中国文学史上著名的寥寥无几.
eg:Shakespeare won laurels in the dramatic world.
The student gained laurels on the football field,as well as in his studies.
Tom won the broad jump,but he had to look to his laurels Getting an A in chemistry almosst cause Mike to rest on his laurels.
希腊罗马神话和《圣经》中的英语典故【7】
7.Swan Song最后杰作;绝笔
Swan Song字面译做“天鹅之歌”,源于希腊成语Kykneion asma.
天鹅,我国古代叫鹄,是一种形状似鹅而体形较大的稀有珍禽,栖息于海滨湖畔,能游善飞,全身白色。因此,英语成语black swan,用以比喻稀有罕见的人或物,类似汉语成语“凤毛麟角”之意。
在古希腊神话中,阿波罗(Apollo)是太阳神、光明之神,由于他多才多艺,又是诗歌与音乐之神,后世奉他为文艺的保护神。天鹅是阿波罗的神鸟,故 常用来比喻文艺。传说天鹅平素不唱歌,而在它死前,必引颈长鸣,高歌一曲,其歌声哀婉动听,感人肺腑。这是它一生中唯一的,也是最后的一次唱歌。因此,西 方各国就用这个典故来比喻某诗人,作家,作曲家临终前的一部杰作,或者是某个演员,歌唱家的最后一次表演。即a last or farewell appearance;the last work before death之意;偶尔也可指某中最后残余的东西。
Swan Song是个古老的成语,源远流长。早在公元前6世纪的古希腊寓言作家伊索(Aisopos)的寓言故事中,就有“天鹅临死才唱歌”的说法。古罗马政治 家、作家西塞罗(Cicero,公元前106-前43)在其《德斯肯伦别墅哲学谈》等论文中,就使用了“天鹅之歌”来比喻临死哀歌。在英国,乔叟,莎士比 亚等伟大诗人、剧作家,都使用过这个成语典故。如:莎翁的著名悲剧《奥噻罗》(othello)中塑造的爱米莉霞的形象,她在生死关头勇敢得站出来揭穿其 丈夫的罪行。她临死时把自己比做天鹅,一生只唱最后一次歌。
eg:All the tickets have been sold for the singer's performance in London this week--the public clearly believes that this will be her swan song
The Tempest was W.Shakespeare's swan song in 1612.
希腊罗马神话和《圣经》中的英语典故【6】
6.A Penelope's Web亦作The Web of Penelope故意拖延的策略;永远做不完的工作
A Penelope's Web或The Web of Penelope,直译为“珀涅罗珀的织物”,典故出自荷马史诗《奥德赛》卷2。
这部史诗的主人公奥德修斯是希腊半岛西南边伊大卡岛(Ithaca)的国王,他有个美丽而忠诚的旗子,名叫珀涅罗珀。奥德修斯随希腊联军远征特洛伊, 十年苦战结束后,希腊将士纷纷凯旋归国。惟独奥德修斯命运坎坷,归途中又在海上漂泊了10年,历尽无数艰险,并盛传他已葬身鱼腹,或者客死异域。正当他在 外流浪的最后三年间,有一百多个来自各地的王孙公子,聚集在他家里,向他的妻子求婚。坚贞不渝的珀涅罗珀为了摆脱求婚者的纠缠,想出个缓宾之策,她宣称等 她为公公织完一匹做寿衣的布料后,就改嫁给他们中的一个。于是,她白天织这匹布,夜晚又在火炬光下把它拆掉。就这样织了又拆,拆了又织,没完没了,拖延时 间,等待丈夫归来。后来,奥德修斯终于回转家园,夫妻儿子合力把那些在他家里宴饮作乐,胡作非为的求婚者一个个杀死,终于夫妻团圆了。
由于这个故事,英语中的Penelope一词成了a chaste woman(贞妇)的同义词,并产生了with a penelope faith(坚贞不渝)这个短语。而A Penelope's Web这个成语比喻the tactics of delaying sth on purposel;the task that can never be finished的意思
eg:Mr Jones made a long speech at the meeting.Everyone else thought it a Penelope's web.
My work is something like the Penelope's web,never done,but ever in hand.
希腊罗马神话和《圣经》中的英语典故【4】
5.Greek Gift(s)阴谋害人的礼物;黄鼠狼拜年,不安好心
Greek Gift(s)直译是“希腊人的礼物”,出自荷马史诗《奥德赛》以及古罗马杰出诗人维吉尔(Publius Virgilius Maro,公元前70-前19年)的史诗《伊尼特》(Aeneis)中关于特洛伊城陷落经过的叙述。
据《奥德赛》卷8记述:许多特洛伊人对如何处置希腊人留下的大木马展开了辩论,“他们有三种主张:有的主张用无情的铜矛刺透中空的木马;有的主张把它 仍到岩石上;有的主张让它留在那里作为京观,来使天神喜悦”。结果是后一说占优势,把那匹木马拖进城里来,终于遭到了亡国之灾。
维吉尔的史诗《伊尼特》,写的是特洛伊被希腊攻陷后,王子伊尼斯从混乱中携家属出走,经由西西里、迦太基到达意大利,在各地漂泊流亡的情况。史诗第2 卷便是伊尼斯关于特洛伊城陷落经过的叙述,其中情节除了模拟荷马史诗的描述外,还做了更详细的补充。当特洛伊人要把大木马拖进城的时候,祭司拉奥孔 (Laocoon)劝说不要接受希腊人留下的东西。他说:“我怕希腊人,即使他们来送礼”这句话后来成了一句拉丁谚语:“Timeo Danaos,et dona ferenteso."(原文的达奈人Danaos,即泛指希腊各部族人)译成英语就是:I fear the Greeks ,even when bringing gifts.其简化形式就是Greek Gifts.可惜特洛伊人不听拉奥孔的警告,把木马作为战利品拖进城里。木马里藏着希腊的精锐部队,给特洛伊人带来了屠杀和灭亡。由此,Greek gift成为一个成语,表示a gift with some sinister purposes of the enemy;one given with intent to harm;a gift sent inorder to murder sb等意思,按其形象意义,这个成语相当与英语的俚谚:When the fox preaches,take care of your geese;也与汉语“黄鼠狼给鸡拜年--不安好心”十分类似
eg:He is always buying you expensive clothes,I'm afraid they are Greek gifts for you.
Comrades,be on guard against the Greek gifts!
To meet Waterloo(倒霉,受毁灭性打击,灭顶之灾)
滑铁卢是一代天骄拿破仑遭受残败的地方。遭遇滑铁卢,对一个人来说,后果不堪设想。无怪据说二战期间,在准备诺曼底反攻时,温斯顿·丘吉尔和随员冒雨 去某地开会,其随员因路滑而摔了一跤,脱口说一句“To meet Waterloo!”丘吉尔竟联想到拿破仑兵败滑铁卢的典故,恼怒地斥责他:“胡说!我要去凯旋门呢!”
It's Greek to me.(我不知道)
英国人一般都不懂希腊语。这句话的直译是:对于我这是希腊语。自然是不明白的意思。
Greek Kalends(幽默,诙谐方式表达的永远不)
Kalends是罗马日历的第一天。古希腊不用罗马日历,永远不会有这一天。
Castle in Spain(西班牙城堡,幻想,梦想。相当于汉语中的空中楼阁)
中世纪某一时期,西班牙是一个颇富浪漫色彩的国家,这句成语是和Castle in air(空中城堡)相齐名的。
Set the Thames on fire(火烧泰晤士河,这是何等伟大的壮举)
但是这句成语经常是反其义应用,指那些人对某事只是夸下海口,而不是真正想去做。
From China to Peru(从中国到秘鲁)
它的意义非常明白,指从世界的这一边到世界的那一边,相当于汉语的远隔重洋。
Between Scylla and Charybdis(锡拉和卡津布迪斯之间———在两个同样危险的事物之间:一个人逃出一种危险,而又落入另一种危险)
锡拉是传说中生活在意大利岩石的怪兽,卡津布迪斯是住在海峡中一端经常产生旋涡的另一个怪兽。水手为了躲避其中一个的危害,而常又落入另一个灾难。意大利这一方的海角叫凯尼斯(Caenys),西西里岛那一方的海角叫皮罗鲁姆(Pelorum)。
Spoil Egyptians(掠夺埃及———迫使敌人提供自己所需要的东西)
源于圣经:上帝答应摩西,埃及人必须借给以色列他们所需要的东西。
Do in Rome as Romans Do(在罗马,就按罗马人的方式办)
和我们的入乡随俗的意思一样。
Carry Coals to Newcastle(把煤送到纽卡斯尔)
把某种东西送到一个人们根本不需要的地方。纽卡斯尔盛产煤,送煤到那里,岂不是多此一举。有趣的是法国也有类似的成语“del'eau a la riviere(送水到大河里)。”
希腊罗马神话和《圣经》中的英语典故【3】
3.Helen of Troy 直译"特洛伊的海伦",源自源自荷马史诗Iliad中的希腊神话故事。
Helen是希腊的绝世佳人,美艳无比,嫁给希腊南部邦城斯巴达国王墨涅俄斯(Menelaus)为妻。后来,特洛伊王子帕里斯奉命出事希腊,在斯巴达国王那里做客,他在爱与美之神阿芙罗狄蒂的帮助下,趁着墨涅俄斯外出之际,诱走海伦,还带走了很多财宝
此事激起了希腊各部族的公愤,墨涅俄斯发誓说,宁死也要夺回海轮,报仇雪恨。为此,在希腊各城邦英雄的赞助下,调集十万大军和1180条战船, 组成了希腊联军,公推墨涅俄斯的哥哥阿枷门农(Agamemnon)为联军统帅,浩浩荡荡,跨海东征,攻打特洛伊城,企图用武力夺回海轮。双方大战10 年,死伤无数,许多英雄战死在沙场。甚至连奥林匹斯山的众神也分成2个阵营,有些支持希腊人,有些帮助特洛伊人,,彼此展开了一场持久的恶斗。最后希腊联 军采用足智多谋的奥德修斯(Odusseus)的“木马计”,里应外合才攻陷了特洛伊。希腊人进城后,大肆杀戮,帕里斯王子也被杀死,特洛伊的妇女、儿童 全部沦为奴隶。特洛伊城被掠夺一空,烧成了一片灰烬。战争结实后,希腊将士带着大量战利品回到希腊,墨涅俄斯抢回了美貌的海轮重返故土。这就是特洛伊战争 的起因和结局。正是由于海轮,使特洛伊遭到毁灭的悲剧,真所谓“倾国倾城”,由此产生了Helen of Troy这个成语。
特洛伊战争的真实性,已为19世纪德国考古学家谢里曼在迈锡尼发掘和考证古代特洛伊古城废墟所证实。至于特洛伊城被毁的真正原因,虽然众说纷 纭,但肯定决不是为了一个美女而爆发这场战争的,与其说是为了争夺海轮而打了起来,毋宁说是为了争夺该地区的商业霸权和抢劫财宝而引起战争的。所谓“特洛 伊的海伦”,实质上是财富和商业霸权的化身。中国历史上也有过“妲己亡商”,“西施沼吴”等传说,以及唐明皇因宠杨贵妃而招致“安史之乱”,吴三桂“冲冠 一怒为红颜”等说法。汉语中有个“倾国倾城”的成语(语出《汉书·外戚传》:‘一顾倾人城,再顾倾人国’。)这里的“倾”字一语双光,既可指美艳非凡,令 人倾倒;也可纸倾覆邦国。其含义与Helen of troy十分近似。
在现代英语中,Helen of Troy这个成语,除了表示a beautiful girl or woman;a beauty who ruins her country等意义外,还可以用来表示a terrible disaster brought by sb or sth you like best的意思。
eg:It is unfair that historians always attribute the fall of kingdoms to Helen of Troy.
She didn't think of the beautiful umbrella bought the day before should become a Helen of Troy in her family.Because of this she and her husband quarreled for a long time.
希腊罗马神话和《圣经》中的英语典故【2】
2.The Heel of Achilles 亦作The Achilles' Heel唯一弱点;薄弱环节;要害
The Heel of Achilles直译是“阿基里斯的脚踵”,是个在欧洲广泛流行的国际性成语。它源自荷马史诗Iliad中的希腊神话故事。
阿基里斯是希腊联军里最英勇善战的骁将,也是荷马史诗Iliad里的主要人物之一。传说他是希腊密耳弥多涅斯人的国王珀琉斯和海神的女儿西蒂斯所生的 儿子。阿基里斯瓜瓜坠地以后,母亲想使儿子健壮永生,把他放在火里锻炼,又捏着他的脚踵倒浸在冥河(Styx)圣水里浸泡。因此阿基里斯浑身象钢筋铁骨, 刀枪不入,只有脚踵部位被母亲的手捏住,没有沾到冥河圣水,成为他的唯一要害。在特洛伊战争中,阿基里斯骁勇无敌,所向披靡,杀死了特洛伊主将,著名英雄 赫克托耳(Hector),而特洛伊的任何武器都无法伤害他的身躯。后来,太阳神阿波罗(Apollo)把阿基里斯的弱点告诉了特洛伊王子帕里斯,阿基里 斯终于被帕里斯诱到城门口,用暗箭射中他的脚踵,负伤而死。
因此,the heel of Achilles,也称the Achilles' heel,常用以表示a weak point in something that is otherwise without fault;the weakest spot等意思。
eg:The shortage of fortitude is his heel of Achilles.
His Achilles' heel was his pride--he would get very angry if anyone criticized his work.
希腊罗马神话和《圣经》中的英语典故 --- 1
1.An Apple of Discord争斗之源;不和之因;祸根
An Apple of Discord直译为“纠纷的苹果”,出自荷马史诗Iliad中的希腊神话故事
传说希腊阿耳戈英雄(Argonaut)珀琉斯(Peleus)和爱琴海海神涅柔斯的女儿西蒂斯(Thetis)在珀利翁山举行婚礼,大摆宴席。
他们邀请了奥林匹斯上(Olympus)的诸神参加喜筵,不知是有意还是无心,惟独没有邀请掌管争执的女神厄里斯(Eris)。这位女神恼羞成 怒,决定在这次喜筵上制造不和。于是,她不请自来,并悄悄在筵席上放了一个金苹果,上面镌刻着“属于最美者”几个字。天后赫拉(Hera),智慧女神雅典 娜(Athena)、爱与美之神阿芙罗狄蒂(Aphrodite),都自以为最美,应得金苹果,获得“最美者”称号。她们争执不下,闹到众神之父宙斯 (Zeus)那里,但宙斯碍于难言之隐,不愿偏袒任何一方,就要她们去找特洛伊的王子帕里斯(Paris)评判。三位女神为了获得金苹果,都各自私许帕里 斯以某种好处:赫拉许给他以广袤国土和掌握富饶财宝的权利,雅典娜许以文武全才和胜利的荣誉,阿芙罗狄蒂则许他成为世界上最美艳女子的丈夫。年青的帕里斯 在富贵、荣誉和美女之间选择了后者,便把金苹果判给爱与美之神。为此,赫拉和雅典娜怀恨帕里斯,连带也憎恨整个特洛伊人。后来阿芙罗狄蒂为了履行诺言,帮 助帕里斯拐走了斯巴达国王墨涅俄斯的王后---绝世美女海伦(Helen),从而引起了历时10年的特洛伊战争。不和女神厄里斯丢下的那个苹果,不仅成了 天上3位女神之间不和的根源,而且也成为了人间2个民族之间战争的起因。因此,在英语中产生了an apple of discord这个成语,常用来比喻any subject of disagreement and contention;the root of the trouble;dispute等意义
这个成语最初为公元2世纪时的古罗马历史学家马克·朱里·尤斯丁(Marcus Juninus Justinus)所使用,后来广泛的流传到欧洲许多语言中去,成为了一个国际性成语。
eg: He throwing us an apple of discord,we soon quarrelled again.
The dispute about inheriting estate formed an apple of discord between them.
This problem seems to be an apple of discord between the Soviet union and the USA.
Installing and Configuring SSL Support
http://java.sun.com/j2ee/1.4/docs/tutorial/doc/Security6.html
What Is Secure Socket Layer Technology?
Secure Socket Layer (SSL) technology allows web browsers and web servers to communicate over a secure connection. In this secure connection, the data that is being sent is encrypted before being sent and then is decrypted upon receipt and before processing. Both the browser and the server encrypt all traffic before sending any data. SSL addresses the following important security considerations.
- Authentication: During your initial attempt to communicate with a web server over a secure connection, that server will present your web browser with a set of credentials in the form of a server certificate. The purpose of the certificate is to verify that the site is who and what it claims to be. In some cases, the server may request a certificate that the client is who and what it claims to be (which is known as client authentication).
- Confidentiality: When data is being passed between the client and the server on a network, third parties can view and intercept this data. SSL responses are encrypted so that the data cannot be deciphered by the third party and the data remains confidential.
- Integrity: When data is being passed between the client and the server on a network, third parties can view and intercept this data. SSL helps guarantee that the data will not be modified in transit by that third party.
To install and configure SSL support on your stand-alone web server, you need the following components. SSL support is already provided if you are using the Application Server. If you are using a different web server, consult the documentation for your product.
- A server certificate keystore (see Understanding Digital Certificates).
- An HTTPS connector (see Using SSL).
To verify that SSL support is enabled, see Verifying SSL Support.
Understanding Digital Certificates
Note: Digital certificates for the Application Server have already been generated and can be found in the directory <
J2EE_HOME
>/domains/domain1/config/
. These digital certificates are self-signed and are intended for use in a development environment; they are not intended for production purposes. For production purposes, generate your own certificates and have them signed by a CA.
To use SSL, an application server must have an associated certificate for each external interface, or IP address, that accepts secure connections. The theory behind this design is that a server should provide some kind of reasonable assurance that its owner is who you think it is, particularly before receiving any sensitive information. It may be useful to think of a certificate as a "digital driver's license" for an Internet address. It states with which company the site is associated, along with some basic contact information about the site owner or administrator.
The digital certificate is cryptographically signed by its owner and is difficult for anyone else to forge. For sites involved in e-commerce or in any other business transaction in which authentication of identity is important, a certificate can be purchased from a well-known certificate authority (CA) such as VeriSign or Thawte.
Sometimes authentication is not really a concern--for example, an administrator may simply want to ensure that data being transmitted and received by the server is private and cannot be snooped by anyone eavesdropping on the connection. In such cases, you can save the time and expense involved in obtaining a CA certificate and simply use a self-signed certificate.
SSL uses public key cryptography, which is based on key pairs. Key pairs contain one public key and one private key. If data is encrypted with one key, it can be decrypted only with the other key of the pair. This property is fundamental to establishing trust and privacy in transactions. For example, using SSL, the server computes a value and encrypts the value using its private key. The encrypted value is called a digital signature. The client decrypts the encrypted value using the server's public key and compares the value to its own computed value. If the two values match, the client can trust that the signature is authentic, because only the private key could have been used to produce such a signature.
Digital certificates are used with the HTTPS protocol to authenticate web clients. The HTTPS service of most web servers will not run unless a digital certificate has been installed. Use the procedure outlined later to set up a digital certificate that can be used by your web server to enable SSL.
One tool that can be used to set up a digital certificate is keytool
, a key and certificate management utility that ships with the J2SE SDK. It enables users to administer their own public/private key pairs and associated certificates for use in self-authentication (where the user authenticates himself or herself to other users or services) or data integrity and authentication services, using digital signatures. It also allows users to cache the public keys (in the form of certificates) of their communicating peers. For a better understanding of keytool
and public key cryptography, read the keytool
documentation at the following URL:
http://java.sun.com/j2se/1.5.0/docs/tooldocs/solaris/key-tool.html
Creating a Server Certificate
A server certificate has already been created for the Application Server. The certificate can be found in the <
J2EE_HOME
>/domains/domain1/config/
directory. The server certificate is in keystore.jks
. The cacerts.jks
file contains all the trusted certificates, including client certificates.
If necessary, you can use keytool
to generate certificates. The keytool
stores the keys and certificates in a file termed a keystore, a repository of certificates used for identifying a client or a server. Typically, a keystore contains one client or one server's identity. The default keystore implementation implements the keystore as a file. It protects private keys by using a password.
The keystores are created in the directory from which you run keytool
. This can be the directory where the application resides, or it can be a directory common to many applications. If you don't specify the keystore file name, the keystores are created in the user's home directory.
To create a server certificate follow these steps:
- Create the keystore.
- Export the certificate from the keystore.
- Sign the certificate.
- Import the certificate into a trust-store: a repository of certificates used for verifying the certificates. A trust-store typically contains more than one certificate. An example using a trust-store for SSL-based mutual authentication is discussed in Example: Client-Certificate Authentication over HTTP/SSL with JAX-RPC.
Run keytool
to generate the server keystore, which we will name keystore.jks
. This step uses the alias server-alias
to generate a new public/private key pair and wrap the public key into a self-signed certificate inside keystore.jks
. The key pair is generated using an algorithm of type RSA, with a default password of changeit
. For more information on keytool
options, see its online help at http://java.sun.com/j2se/1.5.0/docs/tooldocs/solaris/keytool.html
.
Note: RSA is public-key encryption technology developed by RSA Data Security, Inc. The acronym stands for Rivest, Shamir, and Adelman, the inventors of the technology.
From the directory in which you want to create the keystore, run keytool
with the following parameters.
- Generate the server certificate.
- Export the generated server certificate in
keystore.jks
into the fileserver.cer
. - If you want to have the certificate signed by a CA, read Signing Digital Certificates for more information.
- To create the trust-store file
cacerts.jks
and add the server certificate to the trust-store, runkeytool
from the directory where you created the keystore and server certificate. Use the following parameters: - Enter
yes
, and then press theEnter
orReturn
key. The following information displays:
<
JAVA_HOME
>\bin\keytool -genkey -alias server-alias
-keyalg RSA -keypass changeit -storepass changeit
-keystore keystore.jks
When you press Enter, keytool
prompts you to enter the server name, organizational unit, organization, locality, state, and country code. Note that you must enter the server name in response to keytool
's first prompt, in which it asks for first and last names. For testing purposes, this can be localhost
. The host specified in the keystore must match the host identified in the host
variable specified in the <
INSTALL
>/j2eetutorial14/examples/common/build.properties
when running the example applications.
<
JAVA_HOME
>\bin\keytool -export -alias server-alias -storepass changeit -file server.cer -keystore keystore.jks
<
JAVA_HOME
>\bin\keytool -import -v -trustcacerts -alias server-alias -file server.cer -keystore cacerts.jks -keypass changeit -storepass changeit
Information on the certificate, such as that shown next, will display.
<
INSTALL
>/j2eetutorial14/examples/gs 60% keytool -import -v -trustcacerts -alias server-alias -file server.cer -keystore cacerts.jks -keypass changeit -storepass changeit
Owner: CN=localhost, OU=Sun Micro, O=Docs, L=Santa Clara, ST=CA, C=US
Issuer: CN=localhost, OU=Sun Micro, O=Docs, L=Santa Clara, ST=CA, C=US
Serial number: 3e932169
Valid from: Tue Apr 08
Certificate fingerprints:
MD5: 52:9F:49:68:ED:78:6F:39:87:F3:98:B3:6A:6B:0F:90
SHA1: EE:2E:2A:A6:9E:03:9A:3A:1C:17:4A:28:5E:97:20:78:3F:
Trust this certificate? [no]:
Certificate was added to keystore
[Saving cacerts.jks]
tool.html